An Introduction to GraphQL: Benefits and Integration with Next.js Apps
As web and mobile apps evolve, software developers continuously devise innovative methods to enhance communication between application clients and servers. A significant shift in recent years has been the introduction of GraphQL, an open-source query language and runtime designed to optimize API manipulation. Created by Facebook in 2012 and publicly released in 2015, GraphQL addresses the limitations of conventional REST architecture by establishing a novel system that prioritizes declarative, client-centric, and efficient operations.
What is GraphQL?
GraphQL is a query language for your API, and a server-side runtime for executing queries using a type system you define for your data. GraphQL isn't tied to any specific database or storage engine and is instead backed by your existing code and data. A GraphQL service is created by defining types and fields on those types, then providing functions for each field on each type.
Unlike REST, where clients have to make multiple requests to different endpoints to gather all the required data, GraphQL enables clients to retrieve exactly the data they need in a single request. This is achieved through a single endpoint called the "GraphQL endpoint."
In GraphQL, clients define the structure of the data they require using a query language that closely resembles the data they want to retrieve. This eliminates over-fetching (retrieving more data than needed) and under-fetching (not getting all the required data) issues commonly associated with REST APIs. Furthermore, GraphQL supports real-time updates through subscriptions, allowing clients to receive data changes in real-time without continuously polling the server.
Why Use GraphQL?
1. Efficiency: GraphQL minimizes over-fetching and under-fetching of data, resulting in faster and more efficient data retrieval.
2. Flexibility: Clients can request exactly the data they need, which is especially useful for mobile devices with limited bandwidth and resources.
3. Reduced Round Trips: GraphQL's single endpoint reduces the number of round trips between the client and server, improving performance.
4. Versioning: With GraphQL, introducing changes to the API schema doesn't necessarily require versioning, as clients can request only the fields they are interested in.
5. Developer Experience: GraphQL's introspection capabilities allow developers to explore the available data and operations directly, making development and debugging easier.
How to Install GraphQL in Next.js Apps?
Integrating GraphQL into a Next.js application involves a few steps:
- Setting Up a GraphQL Server: You'll need a GraphQL server to handle the queries and mutations from your Next.js app. Popular options include Apollo Server and GraphQL Yoga. These servers can be set up using Node.js.
- Defining the Schema: The schema defines the types of data that can be queried and the structure of the queries themselves. It's the contract between the client and server. You'll define your schema using the GraphQL Schema Definition Language (SDL).
- Creating Resolvers: Resolvers are functions that implement the actual logic for fetching the data based on the queries defined in the schema. Each field in the schema corresponds to a resolver function.
- Integrating with Next.js: To use GraphQL in your Next.js app, you'll need to install the necessary packages, such as
@apollo/client
. This package allows your app to interact with the GraphQL server. - Executing Queries: You can use hooks provided by
@apollo/client
to execute GraphQL queries and mutations within your Next.js components. These hooks manage the communication with the GraphQL server and update your app's state accordingly. - Rendering Data: Once you receive data from the GraphQL server, you can render it in your Next.js components using the retrieved data.
Step by Step Install GraphQL
Step 1: Set Up a New Next.js App
Create a new Next.js app using the following command:
npx create-next-app your-graphql-app
Step 2: Install Dependencies
Navigate to your project directory:
cd your-graphql-app
Install the required dependencies:
npm install @apollo/client graphql
Step 3: Set Up Apollo Client
Open the pages/_app.js
file and configure the Apollo Client to connect to the Pokémon GraphQL server:
import { ApolloProvider, ApolloClient, InMemoryCache } from '@apollo/client';
const client = new ApolloClient({
uri: 'https://graphql-pokeapi.graphcdn.app/', // GraphQL server URL
cache: new InMemoryCache(),
});
function MyApp({ Component, pageProps }) {
return (
<ApolloProvider client={client}>
<Component {...pageProps} />
</ApolloProvider>
);
}
export default MyApp;
We are utilizing an unofficial Pokémon GraphQL server for our GraphQL operations. You can refer to its documentation at https://graphql-pokeapi.vercel.app/ and explore its live GraphQL Playground at https://graphql-pokeapi.graphcdn.app/.
Step 4: Create a GraphQL Query
Create a new file, pages/index.js
, and add the following code to fetch a list of Pokémon names from the GraphQL server:
import { useQuery, gql } from '@apollo/client';
const GET_POKEMONS = gql`
query pokemons($limit: Int, $offset: Int) {
pokemons(limit: $limit, offset: $offset) {
count
next
previous
status
message
results {
url
name
image
}
}
}
`;
const gqlVariables = {
limit: 2,
offset: 1,
};
function Home() {
const { loading, error, data } = useQuery(GET_POKEMONS, {
variables: gqlVariables,
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const pokemonNames = data?.pokemons.results.map(pokemon => pokemon.name);
return (
<div>
<h1>Pokémon Names</h1>
<ul>
{pokemonNames.map((name, index) => (
<li key={index}>{name}</li>
))}
</ul>
</div>
);
}
export default Home;
Step 5: Start the Development Server
Run the development server:
npm run dev
Visit http://localhost:3000
in your browser, and you should see a list of Pokémon names fetched from the GraphQL server.
Conclusion
In conclusion, the rise of GraphQL marks a significant advancement in the realm of API development. Its efficiency, flexibility, reduced round trips, and improved developer experience set it apart from traditional REST APIs. By allowing clients to request precisely the data they need through a single endpoint, GraphQL streamlines data retrieval and consumption, resulting in enhanced performance and responsiveness.
Reference
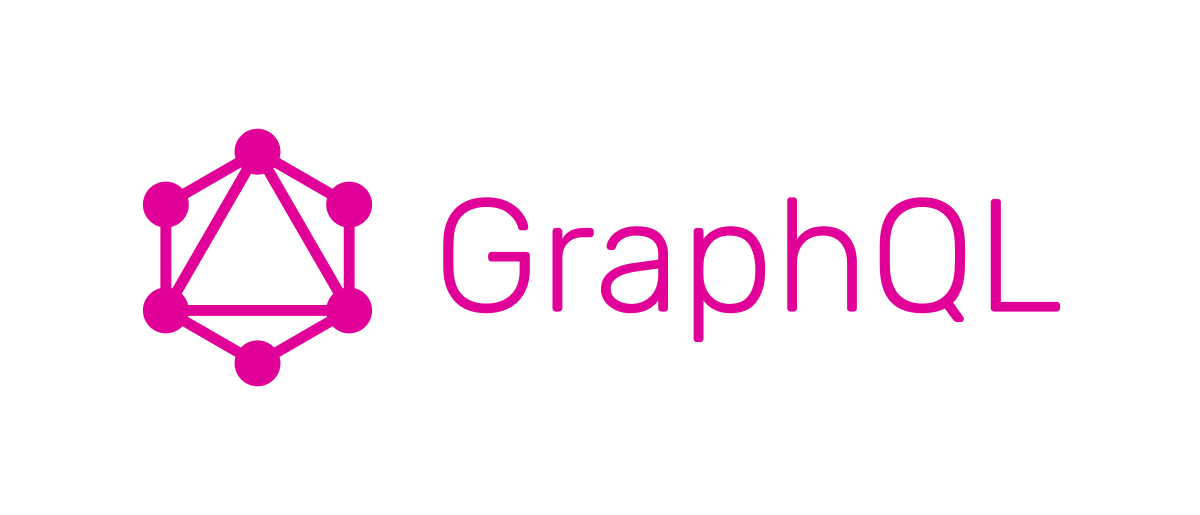